RevenueCat Flutter SDK adds web support (beta)
Flutter developers can now use RevenueCat to manage subscriptions across web, mobile, and desktop platforms.
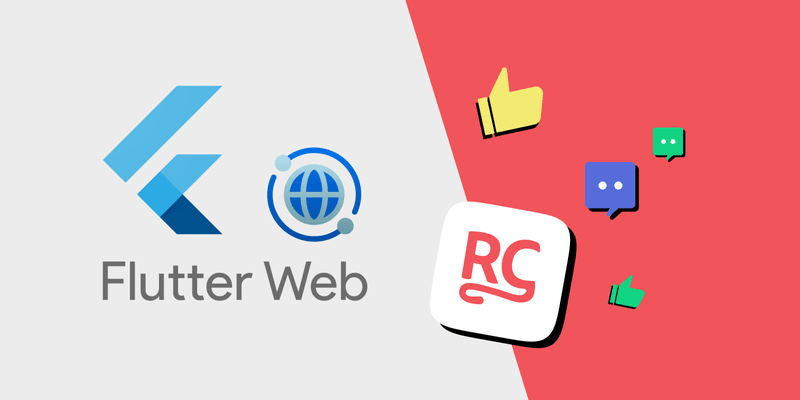
Quick summary: The RevenueCat Flutter SDK now includes beta support for Flutter Web. Developers can configure web entitlements, set up web-specific products and offerings, and use a new Web Billing API key to integrate RevenueCat’s purchase flow across platforms including iOS, Android, macOS, and now the web.
One of Flutter’s biggest strengths is its ability to compile on mobile (iOS, Android), desktop (macOS, Windows, Linux), embedded platforms, and even the web.
With a single code base and the help of the RevenueCat SDK, developers can release a seamless multi-platform experience for their customers, allowing them to access their entitlements across devices and platforms.
Until now, the RevenueCat Flutter SDK hasn’t supported Flutter web apps. This meant that Flutter developers wanting to release a web app would have to manually fetch entitlements and subscription status and integrate a custom web checkout experience.
Flutter developers can now target the web platform, and the RevenueCat SDK will work seamlessly, just as it already does on iOS, Android, and macOS.
Here’s how to set up your Flutter project for web support (with a few beta limitations).
Step 1: Configure RevenueCat Web
Select your project in the RevenueCat Dashboard, and add the RevenueCat Web Billing payment provider.

You’ll then need to connect your Stripe account, set the default currency, and add app and brand information that will appear in the web checkout experience. Detailed instructions for setting this up are available here.
Step 2: Configure Web Products
Unlike mobile platforms, you can create web products directly in the RevenueCat dashboard. Create the products, add them to your offerings, and attach them to the relevant entitlements.
Step 3: Upgrade the RevenueCat Flutter SDK
Open your Flutter project and update your RevenueCat Flutter SDK dependency in your pubspec.yaml file to 9.0.0-beta.1.
1dependencies:
2
3 purchases_flutter: ^9.0.0-beta.1
Then, fetch the updated package.
1flutter pub get
Step 4: Enable Web in Your Flutter Project
Ensure your Flutter app can build for web. Run the following command in your Flutter project and ensure all dependencies in your pubspec.yaml file also support web.
1flutter create . --platforms web
Step 5: Configure the Web Platform in the SDK
Using your new RevenueCat Web API key from the dashboard, modify your SDK configuration code to provide this API key when the platform is web.
1import 'dart:io' show Platform;
2
3//...
4
5Future<void> initPlatformState() async {
6
7 await Purchases.setLogLevel(LogLevel.debug);
8
9 PurchasesConfiguration configuration;
10
11 if (kIsWeb) {
12
13 configuration = PurchasesConfiguration(<public_rc_web_billing_api_key>);
14
15 } else if (Platform.isAndroid) {
16
17 configuration = PurchasesConfiguration(<public_google_api_key>);
18
19 } else if (Platform.isIOS) {
20
21 configuration = PurchasesConfiguration(<public_apple_api_key>);
22
23 }
24
25 await Purchases.configure(configuration);
26
27}
Step 6: Test the Web Purchase Flow
As with mobile and desktop platforms, you can continue to call purchasePackage on your paywall to initiate the purchase flow.
Instead of the native in-app purchase checkout, you’ll now see a web-specific checkout experience fully handled by RevenueCat.

Step 7: Handle Beta Limitations
The following functionality isn’t yet supported in the RevenueCat Flutter SDK when the platform is web:
- Setting attributes
- Product operations (get/purchase products)
- Restoring purchases (Purchases can be restored using Web Billing’s built-in mechanisms)
If your mobile app uses these methods, add a web platform check and handle them appropriately.
1Future unsupportedMethodOnWeb() {
2
3 if (kIsWeb) return; // or throw an error
4
5 ...
6
7 // continue with non-web functionality
8
9}
Please note that Paywalls, Customer Center, and other features from the purchases_ui_flutter package aren’t yet available on the web.
Build once, deploy everywhere
We’re big fans of Flutter here at RevenueCat and are happy to support Flutter developers expanding their apps to the web.
Please share any feedback or bugs with our team during the beta period here. We’re working hard to bring all SDK functionality to Flutter Web soon. We hope you enjoy this new capability!
You might also like
- Blog post
Google I/O 2025: Monetization & Play Console Highlights (and the case of the missing Billing Library 8)
Key monetization takeaways from Google I/O 2025: Play Console redesign, subscription bundles, churn‑busting tools, and a peek at Billing Library 8
- Blog post
How to monetize Android apps with ad-free subscriptions using RevenueCat
A step-by-step approach to monetizing Android users with an optional ad-free upgrade.
- Blog post
Ensure public interface reliability: Tracking API compatibility for Android and Kotlin
This article explores how to ensure public API reliability by tracking compatibility changes, by exploring the RevenueCat SDK.